1. Download STS, there are two ways to use spring tools. First, eclipse with its plugins,
go to eclipse marketing place. I didn’t recommend using raw eclipse with spring plugins,
because during I develop, I find eclipse with plugin may fail to work.sometime, it may reinstall every time when eclipse restart.
I highly recommend to download STS whole toolset form https://spring.io/tools/sts. Once it is downloaded and unpacked, it will be used as eclipse.
2. Maven is mainly project management tools. Two important concept.
Parent POM and Child POM, in parent project, it has pom.xml which will contains child project pom.xml as module, while child project will also reference parent project in its POM.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
| <?xml version="1.0" encoding="UTF-8"?>
<project>
...
<groupId>org.springframework.samples</groupId>
<artifactId>spring-petclinic-angular1</artifactId>
<version>2.0.0-M3</version>
<name>Spring Petclinic :: Parent POM</name>
<packaging>pom</packaging>
<modules>
<module>spring-petclinic-client</module>
<module>spring-petclinic-server</module>
</modules>
...
</project> |
<?xml version="1.0" encoding="UTF-8"?>
<project>
...
<groupId>org.springframework.samples</groupId>
<artifactId>spring-petclinic-angular1</artifactId>
<version>2.0.0-M3</version>
<name>Spring Petclinic :: Parent POM</name>
<packaging>pom</packaging>
<modules>
<module>spring-petclinic-client</module>
<module>spring-petclinic-server</module>
</modules>
...
</project>
1
2
3
4
5
6
7
8
9
| <project>
...
<parent>
<groupId>org.springframework.samples</groupId>
<artifactId>spring-petclinic-angular1</artifactId>
<version>2.0.0-M3</version>
</parent>
...
</project> |
<project>
...
<parent>
<groupId>org.springframework.samples</groupId>
<artifactId>spring-petclinic-angular1</artifactId>
<version>2.0.0-M3</version>
</parent>
...
</project>
3. Create parent project
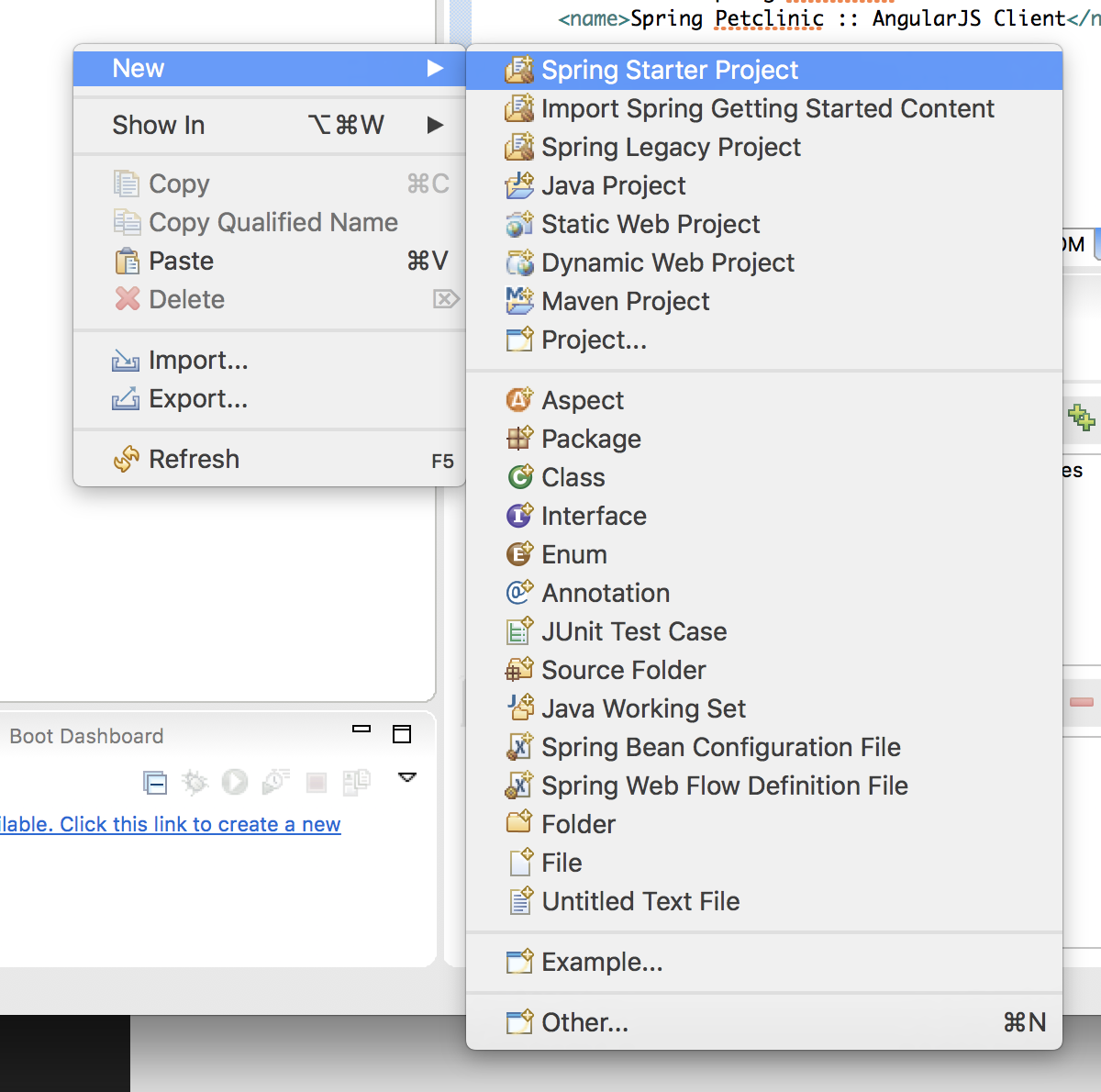
<1>.choose ‘Spring Start Project’, click ‘next’ following by wizard filling with information.
<2>.delete all folders, only keep two folders ‘spring-petclinic-client’ and ‘spring-petclinic-server’ for child projects
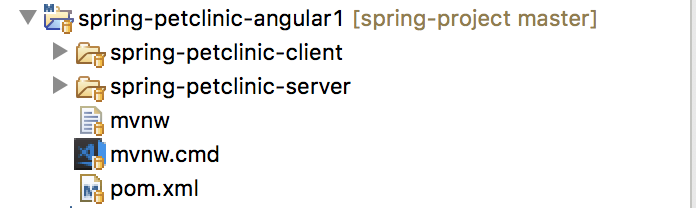
4. Create child frontend project
<1> choose ‘Spring Start Project’, click ‘next’ following by wizard filling with information.
<2> delete ‘src/main/java’, ‘src/main/resources’, ‘src/test/java’
<3> add bower.json, glupfile.js, package.json and keep src as resource.
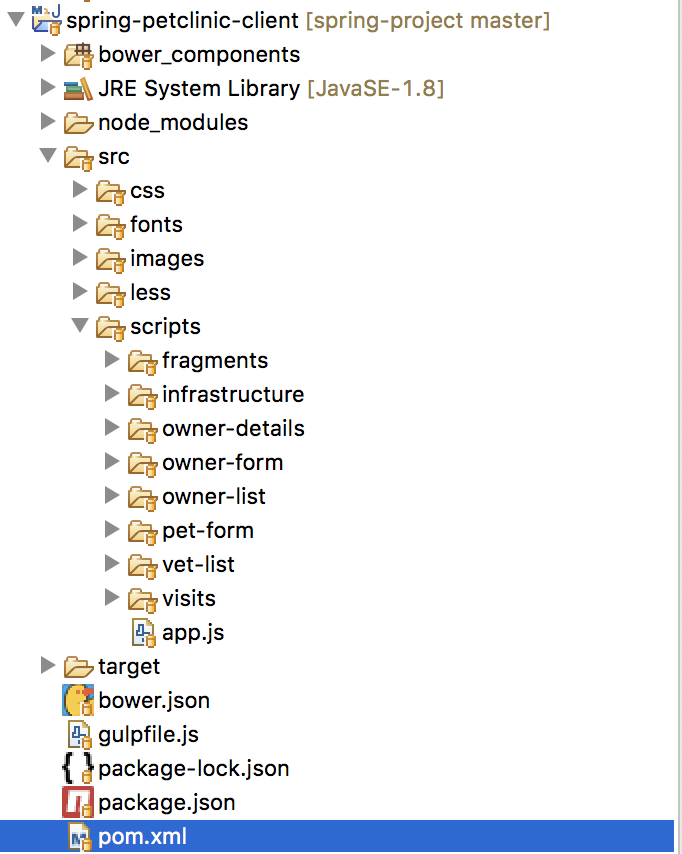
5. create child backend project
<1> choose ‘Spring Start Project’, click ‘next’ following by wizard filling with information.
for backend, project is OK to keep original structure.
restful controller will map url to method.
@PostMapping(“/owners/{ownerId}/pets/{petId}/visits”)
@ResponseStatus(HttpStatus.NO_CONTENT)
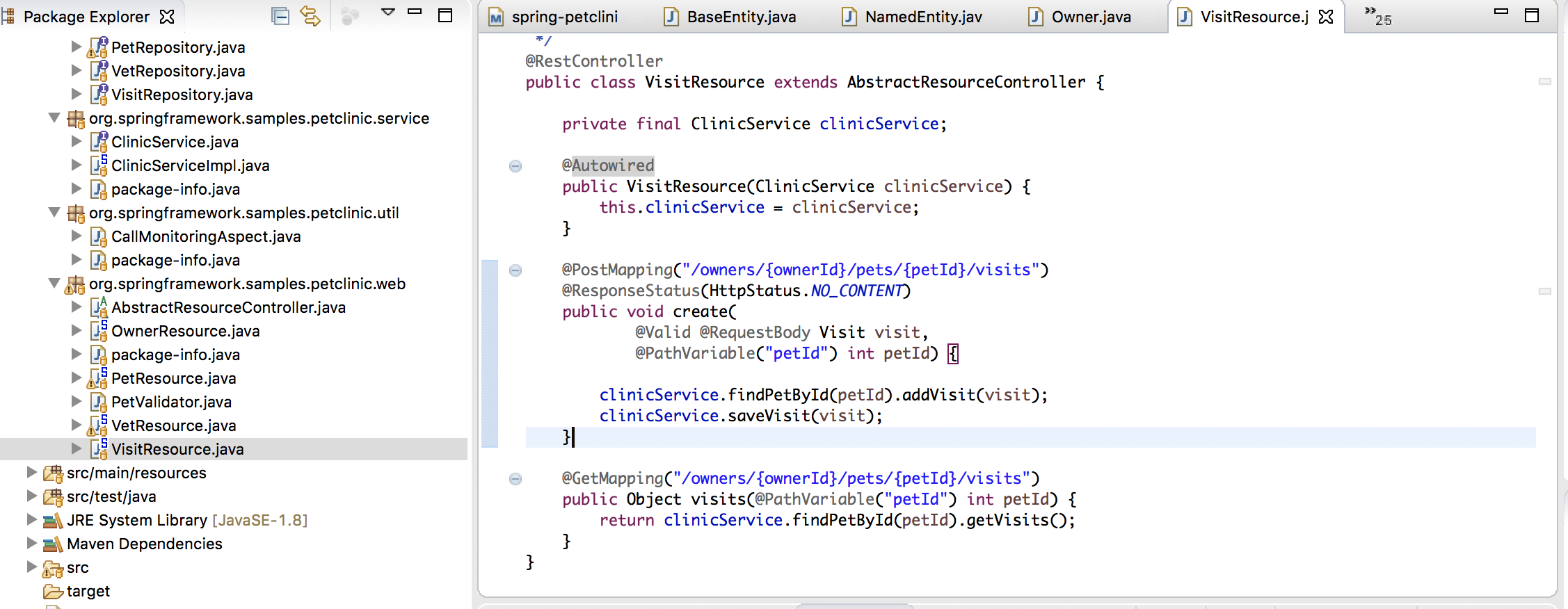
6. run ‘mvn package -DskipTest’ on parent project folder to build all project.
Now we finish all project structure creating process.
for more code, https://github.com/emacslisp/spring-project/tree/master/spring-petclinic-angular1